Java Constructors and Types
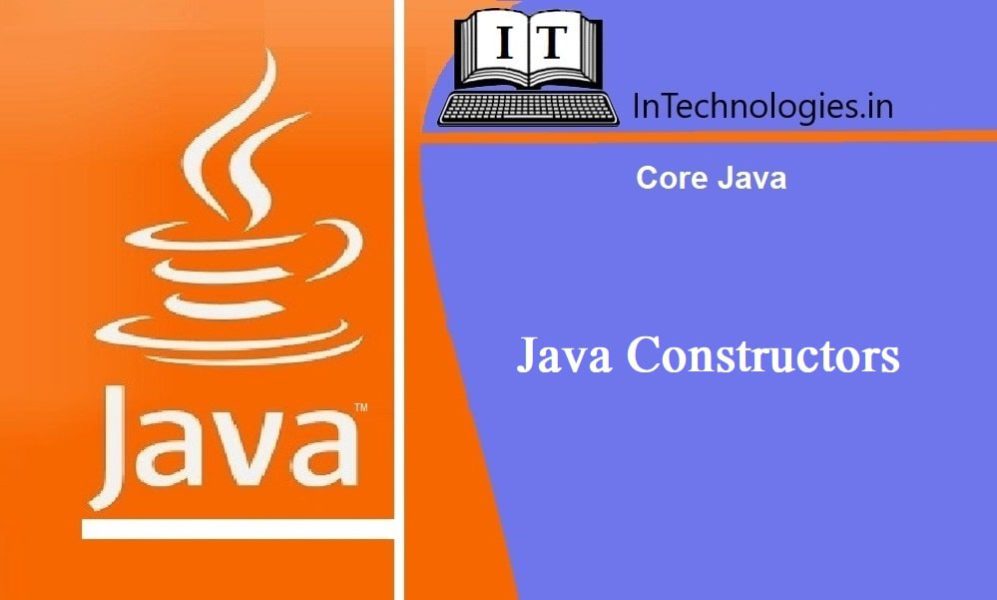
Table of Contents
Constructor is a block of code that initializes the newly created object and allocate the memory for object. All classes have constructors by default. A Java constructors cannot be final, abstract, static and synchronized.
When object is created, the constructor initialize the object with there default values. It has the same name as class name and is syntax is similar to a method. However, constructors have no explicit return type.
Example
class Employee { // No argument constructor Employee() { } }
Types of constructors in java :-
1. No argument Constructor
A constructor that has no arguments parameter is known as default constructor. If in a class, there is no define a constructor in a class, then java compiler creates default constructor(with no arguments) for the class.
Example
// No argument/parameter Constructor Public class MyClass { Int numvalue; MyClass() { numvalue = 10; } }
2. Parameterized Constructors
A Constructor that takes arguments / parameters is known as Parameterized or overloaded constructor. Constructor name is same as class name.
Example
// Parameterized constructor. class Employee { int numvalue; // Below defines the parameterized constructor Employee(int parameterValue) { numvalue = parameterValue; } }
Question :-
- What are Parameterized Constructors?
- What is No argument Constructor?
- What is a Constructor?
- Do we have Copy Constructor in Java?
- Can we call sub class constructor from super class constructor?
- What are private constructors and where are they used?
- Do we have destruction in Java?
- When do we need Constructor Overloading?
0 Comments