Java Basic Datatypes
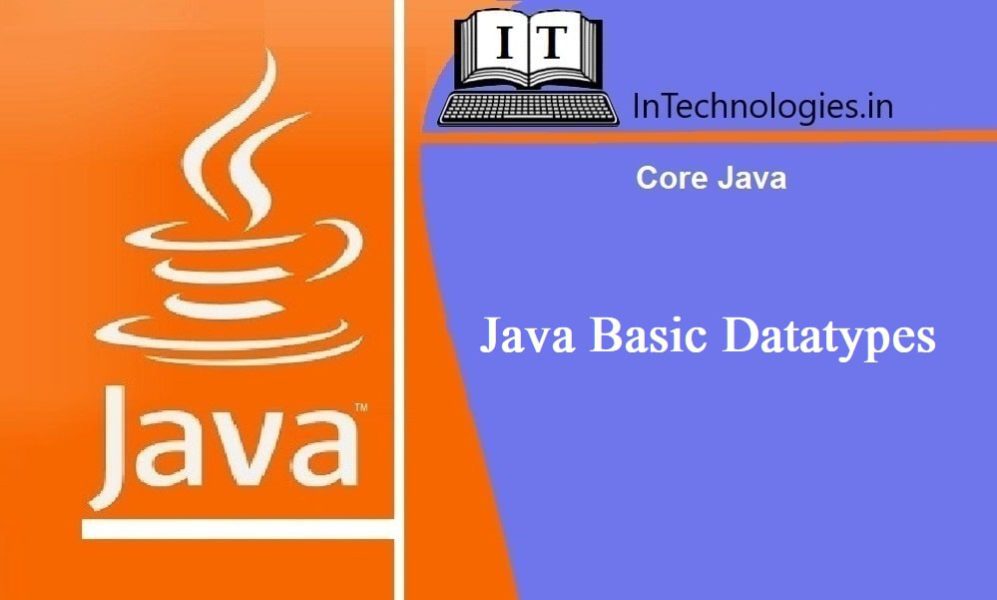
Table of Contents
Java Datatypes specify the values and different sizes that can be stored in the variable.
Two types of data types in Java :-
- Primitive data types : Eight primitive data types available in java. The primitive data types include byte, short, int, long, float and double, boolean, char.
- Non-primitive data types : The non-primitive data types include Arrays, Classes, And Interfaces.
Two data types available in Java :−
- Primitive Data Types
- Reference/Object Data Types
Primitive Data Types
There are eight primitive datatypes supported by Java. Primitive datatypes are predefined by the language and are designated by a keyword. Let us now look into the eight primitive data types size, range and default values.
Type | Default Size | Range | Default Value | Example |
---|---|---|---|---|
byte | 1 byte | -128 to 127 | 0 | byte a = 10, byte b = -20 |
short | 2 bytes | -32,768 to 32,767 | 0 | short a = 10000, short b = -5000 |
int | 4 bytes | -2,147,483,648 to 2,147,483, 647 | 0 | int a = 100000, int b = -200000 |
long | 8 bytes | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | 0 | long a = 100000L, long b = -200000L |
float | 4 bytes | approximately ±3.40282347E+38F (6-7 significant decimal digits) Java implements IEEE 754 standard | 0.0f | float a = 234.5f |
double | 8 bytes | approximately ±1.79769313486231570E+308 (15 significant decimal digits) | 0.0d | double a = 12.3 |
char | 2 byte | 0 to 65,536 (unsigned) | ‘\u0000’ | char letterA = ‘A’ |
boolean | 1 bit | true or false | false | boolean flag = false |
Reference/Object Datatypes
- Reference variables are created using defined constructors of classes. They are used to access objects. These variables are declared to be of a particular type which cannot be changed. For example, Employee, Puppy, etc.
- Class objects and variety of array variables come under reference datatype.
- Default value of any reference variable is null.
- It can be used to refer any object of the declared type or any compatible type.
- Example: Animal animal = new Animal(“giraffe”);
Escape Sequences
Java language supports few special escape sequences for char and String literals as well. They are −
Escape Sequences | Character represented |
---|---|
\n | Newline (0x0a) |
\r | Carriage return (0x0d) |
\f | Formfeed (0x0c) |
\b | Backspace (0x08) |
\s | Space (0x20) |
\t | tab |
\” | Double quote |
\’ | Single quote |
\\ | backslash |
\ddd | Octal character (ddd) |
\uxxxx | Hexadecimal UNICODE character (xxxx) |
Question :-
- what are Primitive data type in java?
- What are long data type in Java?
- How does Java pass primitive variables to methods – by value or by reference?
0 Comments